Naming Convention in C++ Programming Language
C++ Basic Concepts
In this lesson, we will learn about the Naming Convention in the C++ programming language. We will go through the rules of naming, along with examples and a quiz on it.
What is Naming Conventions
In C++ programming, naming conventions are set of rules for choosing the valid name to be used for variable in a C++ program.
Naming Conventions rules for Variables are:
- It should begin with an alphabet.
- There may be more than one alphabet, but without any spaces between them.
- Digits may be used but only after alphabet.
- No special symbol can be used except the underscore (_) symbol. When multiple words are needed, an underscore should separate them.
- No keywords or command can be used as a variable name.
- All statements in C++ language are case sensitive. Thus a variable A (in uppercase) is considered different from a variable declared a (in lowercase).
Now let's see some examples for more understanding.
Example 1
It should begin with an alphabet.
x // x is a valid variable name because it starts with an alphabet x
Example 2
There may be more than one alphabet, but without any spaces between them.
total // total is a valid variable name as there is no space between alphabets
Example 3
Digits may be used but only after alphabet.
ar15 // ar15 is a valid variable name as digits have been used after the alphabet
a6b2 // a6b2 is a valid variable name as digits have been used after the alphabet
Example 4
No special symbol should be present within the variable name except underscore _.
total_cost // total_cost is a valid variable name as there is an underscore
total cost // total cost is an invalid variable name as there is a space
total-cost // total-cost is an invalid variable name as there is a hyphen
total$ // total$ is an invalid variable name as there is a dollar symbol
Example 5
No keywords or command can be used as a variable name.
for // here for is an invalid variable name because it is a keyword in C++
if // if is an invalid variable name because it is a keyword in C++
case // case is an invalid variable name because it is a keyword in C++
const // const is an invalid variable name because it is a keyword in C++
Example 6
All statements in C++ language are case sensitive. Thus a variable A (in uppercase) is considered different from a variable declared a (in lowercase).
a // a is a valid variable written in lowercase
A // A is a valid variable written in uppercase so both are different variables
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
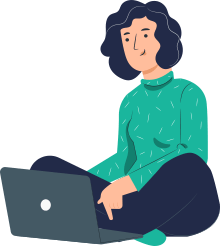